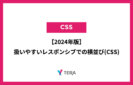
JavaScriptでサイト内にリアルタイムの時計を設置する方法
カテゴリ:WEB制作
タグ:
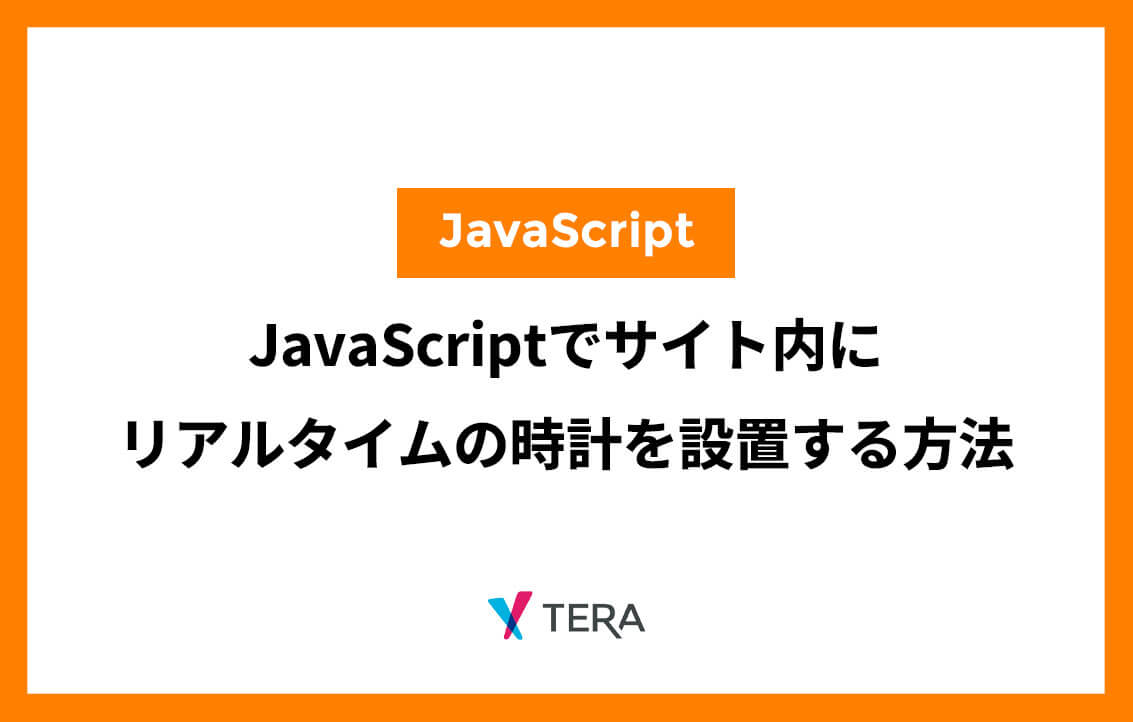
年末のカウントダウンや商品やイベントの開催時刻を知らせる際に使用するJavaScriptでサイト内にリアルタイムの時計を設置する方法を紹介します。
コードを紹介
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>リアルタイム時計</title> </head> <body> <span class="year"> <span class="year_num"></span>年 </span> <span class="date"> <span class="date_num"></span>月 <span class="date_num"></span>日 </span> <span class="time"> <span class="time_num"> 00:00:00 </span> </span> <script> document.addEventListener("DOMContentLoaded", function () { const yearElement = document.querySelector(".year_num"); const monthElement = document.querySelectorAll(".date .date_num")[0]; const dayElement = document.querySelectorAll(".date .date_num")[1]; const timeElement = document.querySelector(".time .time_num"); function updateClock() { const now = new Date(); const year = now.getFullYear(); const month = now.getMonth() + 1; const day = now.getDate(); const hours = String(now.getHours()).padStart(2, "0"); const minutes = String(now.getMinutes()).padStart(2, "0"); const seconds = String(now.getSeconds()).padStart(2, "0"); yearElement.textContent = year; monthElement.textContent = month; dayElement.textContent = day; timeElement.textContent = `${hours}:${minutes}:${seconds}`; } updateClock(); setInterval(updateClock, 1000); }); </script> </body> </html>
コードを解説
HTML
JavaScriptで取得した日時を出力するためにそれぞれクラス名を付与しておきます。
<span class="year"> <span class="year_num"></span>年 </span> <span class="date"> <span class="date_num"></span>月 <span class="date_num"></span>日 </span> <span class="time"> <span class="time_num"> 00:00:00 </span> </span>
JavaScript
読み込みが完了したら実行します。
document.addEventListener("DOMContentLoaded", function () { });
HTMLで出力用に振っているクラス名を指定して取得していきます。
const yearElement = document.querySelector(".year_num"); const monthElement = document.querySelectorAll(".date .date_num")[0]; const dayElement = document.querySelectorAll(".date .date_num")[1]; const timeElement = document.querySelector(".time .time_num");
時間の取得とセットを行うための関数を書いてきます。
『new Date()』で時間が取得できます。
年 | ○○○.getFullYear(); |
月 | ○○○.getMonth() + 1; |
日付 | ○○○.getDate(); |
時間 | String(○○○.getHours()).padStart(2, “0”); |
分 | String(○○○.getMinutes()).padStart(2, “0”); |
秒 | String(○○○.getSeconds()).padStart(2, “0”); |
const now = new Date(); const year = now.getFullYear(); const month = now.getMonth() + 1; const day = now.getDate(); const hours = String(now.getHours()).padStart(2, "0"); const minutes = String(now.getMinutes()).padStart(2, "0"); const seconds = String(now.getSeconds()).padStart(2, "0");
次にそれぞれを『.textContent』で出力します。
yearElement.textContent = year; monthElement.textContent = month; dayElement.textContent = day; timeElement.textContent = `${hours}:${minutes}:${seconds}`;
ページの読み込み時に一回実行させます。
updateClock();
以降は1秒ごとにupdateClock関数を実行し、リアルタイム更新を行っています。
setInterval(updateClock, 1000);
まとめ
以上、『JavaScriptでサイト内にリアルタイムの時計を設置する方法』でした。キャンペーンサイト、商品サイトなどで販売日までのにタイムを表示したりするのに活用できると思います。ぜひ参考にしてみてください。