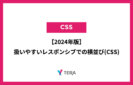
Appleの製品比較のような表をJavascriptで作成する方法
カテゴリ:WEB制作
タグ:
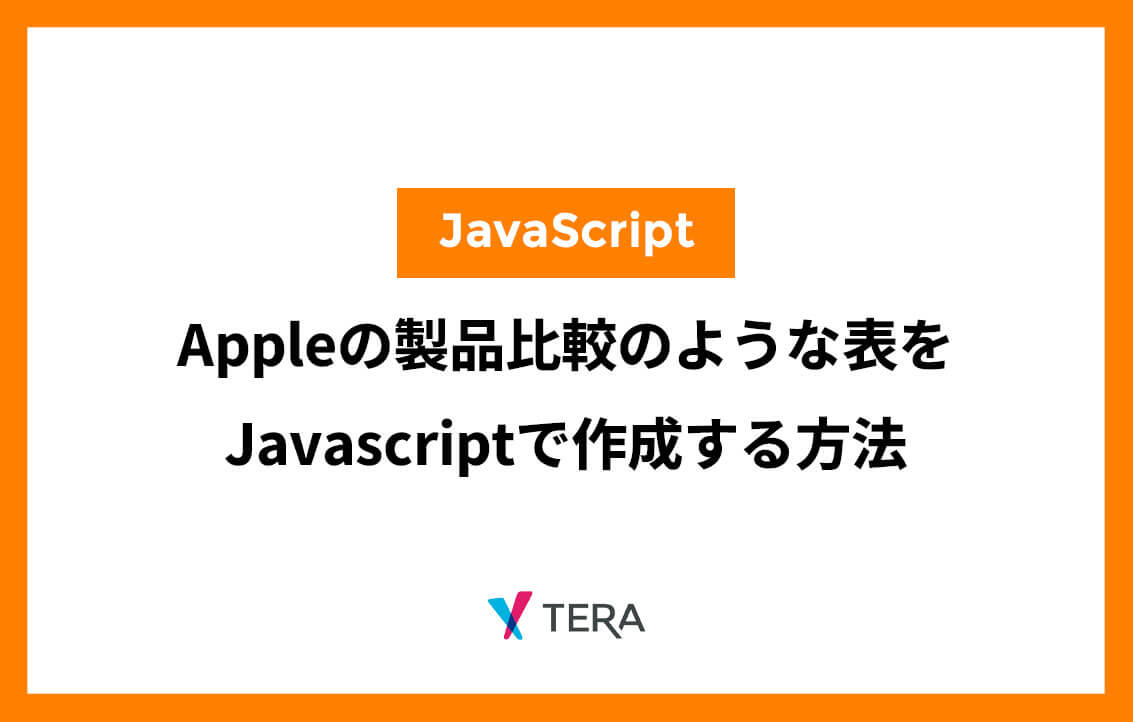
今回はAppleのiPhoneのモデル比較のような表を作成する方法を紹介します。
製品情報はJSONでから読み込みますが、今回は外部ファイルとして読み込まず、Javascriptに直接埋め込みます。
コードを紹介
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>製品比較</title> </head> <body> <ul> <li> <select id="product1"></select> </li> <li> <select id="product2"></select> </li> </ul> <table class="comparison_table" border="1"> <tr> <th>画面サイズ</th> <td id="size1"></td> <td id="size2"></td> </tr> <tr> <th>高さ</th> <td id="height1"></td> <td id="height2"></td> </tr> <tr> <th>幅</th> <td id="width1"></td> <td id="width2"></td> </tr> <tr> <th>厚さ</th> <td id="heavy1"></td> <td id="heavy2"></td> </tr> <tr> <th>重量</th> <td id="weight1"></td> <td id="weight2"></td> </tr> </table> <script> document.addEventListener("DOMContentLoaded", function () { const productData = [ {"name": "iPhone16", "size": "6.1インチ", "height": "147.6mm", "width": "71.6mm", "heavy": "7.8mm", "weight": "170g"}, {"name": "iPhone15", "size": "6.1インチ", "height": "147.6mm", "width": "71.6mm", "heavy": "7.8mm", "weight": "171g"}, {"name": "iPhone14", "size": "6.1インチ", "height": "146.7mm", "width": "71.5mm", "heavy": "7.8mm", "weight": "172g"}, {"name": "iPhone13", "size": "6.1インチ", "height": "146.7mm", "width": "71.5mm", "heavy": "7.65mm", "weight": "173g"}, {"name": "iPhone12", "size": "6.1インチ", "height": "146.7mm", "width": "71.5mm", "heavy": "7.65mm", "weight": "162g"}, {"name": "iPhone11", "size": "6.1インチ", "height": "150.9mm", "width": "75.7mm", "heavy": "8.3mm", "weight": "194g"} ]; const select1 = document.getElementById("product1"); const select2 = document.getElementById("product2"); function populateSelectOptions() { select1.innerHTML = ""; select2.innerHTML = ""; productData.forEach((product, index) => { const option1 = document.createElement("option"); option1.value = product.name; option1.textContent = product.name; if (index === 0) option1.selected = true; select1.appendChild(option1); const option2 = document.createElement("option"); option2.value = product.name; option2.textContent = product.name; if (index === 1) option2.selected = true; select2.appendChild(option2); }); updateComparisonTable(); } function updateComparisonTable() { const product1 = productData.find(p => p.name === select1.value); const product2 = productData.find(p => p.name === select2.value); if (product1 && product2) { document.getElementById("size1").textContent = product1.size; document.getElementById("size2").textContent = product2.size; document.getElementById("height1").textContent = product1.height; document.getElementById("height2").textContent = product2.height; document.getElementById("width1").textContent = product1.width; document.getElementById("width2").textContent = product2.width; document.getElementById("heavy1").textContent = product1.heavy; document.getElementById("heavy2").textContent = product2.heavy; document.getElementById("weight1").textContent = product1.weight; document.getElementById("weight2").textContent = product2.weight; } } select1.addEventListener("change", updateComparisonTable); select2.addEventListener("change", updateComparisonTable); populateSelectOptions(); }); </script> </body> </html>
コードを解説
HTML
セレクトボックス選択用のパーツをリストタグで作成、それぞれにセレクトボックスを設置し、Javascriptの発火用にidを振っておきます。
<ul> <li> <select id="product1"></select> </li> <li> <select id="product2"></select> </li> </ul>
次に表示用のテーブルタグを作成します。
値の箇所にはそれぞれidを振っておきます。
<table border="1"> <tr> <th>画面サイズ</th> <td id="size1"></td> <td id="size2"></td> </tr> <tr> <th>高さ</th> <td id="height1"></td> <td id="height2"></td> </tr> <tr> <th>幅</th> <td id="width1"></td> <td id="width2"></td> </tr> <tr> <th>厚さ</th> <td id="heavy1"></td> <td id="heavy2"></td> </tr> <tr> <th>重量</th> <td id="weight1"></td> <td id="weight2"></td> </tr> </table>
Javascript
読み込みが終わってから実行します。
document.addEventListener("DOMContentLoaded", function() {});
Jsonデータを登録します。
Jsonデータはキーと値、複数挿入する場合はカンマ区切りで設置します。
以下では変数に格納しています。
const productData = [ {"name": "iPhone16", "size": "6.1インチ", "height": "147.6mm", "width": "71.6mm", "heavy": "7.8mm", "weight": "170g"}, {"name": "iPhone15", "size": "6.1インチ", "height": "147.6mm", "width": "71.6mm", "heavy": "7.8mm", "weight": "171g"}, {"name": "iPhone14", "size": "6.1インチ", "height": "146.7mm", "width": "71.5mm", "heavy": "7.8mm", "weight": "172g"}, {"name": "iPhone13", "size": "6.1インチ", "height": "146.7mm", "width": "71.5mm", "heavy": "7.65mm", "weight": "173g"}, {"name": "iPhone12", "size": "6.1インチ", "height": "146.7mm", "width": "71.5mm", "heavy": "7.65mm", "weight": "162g"}, {"name": "iPhone11", "size": "6.1インチ", "height": "150.9mm", "width": "75.7mm", "heavy": "8.3mm", "weight": "194g"} ];
次に製品を選択するセレクトボックスを取得します。
const select1 = document.getElementById("product1"); const select2 = document.getElementById("product2");
セレクトボックスを初期化して、製品リストを追加する関数を書いていきます。
function populateSelectOptions() { }
以下でHTMLを空にして初期化します。
select1.innerHTML = ""; select2.innerHTML = "";
Jsonデータをループ処理させて商品名を取得します。
productData.forEach((product, index) => { });
セレクトボックスは二つあるため二つ同じ処理を行います。
一つ目のセレクトボックスだけ解説します。
セレクトボックスのoptionタグを生成します。
const option1 = document.createElement("option");
optionタグの値とテキストを以下で設定します。
option1.value = product.name; option1.textContent = product.name;
最初のオプションタグの処理のみ初期値として設定します。
二つ目のセレクトボックスは2番目のモデルを追加します。
if (index === 0) option1.selected = true;
セレクトボックス内にoptionタグを生成します。
select1.appendChild(option1);
表の更新をする関数を発火させます。
表の更新を行う処理を書いていきます。
function updateComparisonTable() { }
『.find』を使用してJsonデータ内のネーム属性とセレクトボックスのバリュー値を比較して対象の製品モデルの列を取得します。
『iPhone16』の場合はJsonの一列目が取得されます。
セレクトボックスは二つあるため二回処理を行います。
const product1 = productData.find(p => p.name === select1.value); const product2 = productData.find(p => p.name === select2.value);
データが取得できた場合は、以下で表の値を書き換えます。
if (product1 && product2) { document.getElementById("size1").textContent = product1.size; document.getElementById("size2").textContent = product2.size; document.getElementById("height1").textContent = product1.height; document.getElementById("height2").textContent = product2.height; document.getElementById("width1").textContent = product1.width; document.getElementById("width2").textContent = product2.width; document.getElementById("heavy1").textContent = product1.heavy; document.getElementById("heavy2").textContent = product2.heavy; document.getElementById("weight1").textContent = product1.weight; document.getElementById("weight2").textContent = product2.weight; }
最後にセレクトボックスを変更した時に表の更新を発火するタグと初期化を行う処理を記載します。
select1.addEventListener("change", updateComparisonTable); select2.addEventListener("change", updateComparisonTable); populateSelectOptions();
以上で実装完了です。
まとめ
以上、『Appleの製品比較のような表をJavascriptで作成する方法』でした。