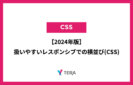
セレクトボックスの選択肢に合わせて入力項目を増やす、別サイトに飛ばすの実装方法
カテゴリ:WEB制作
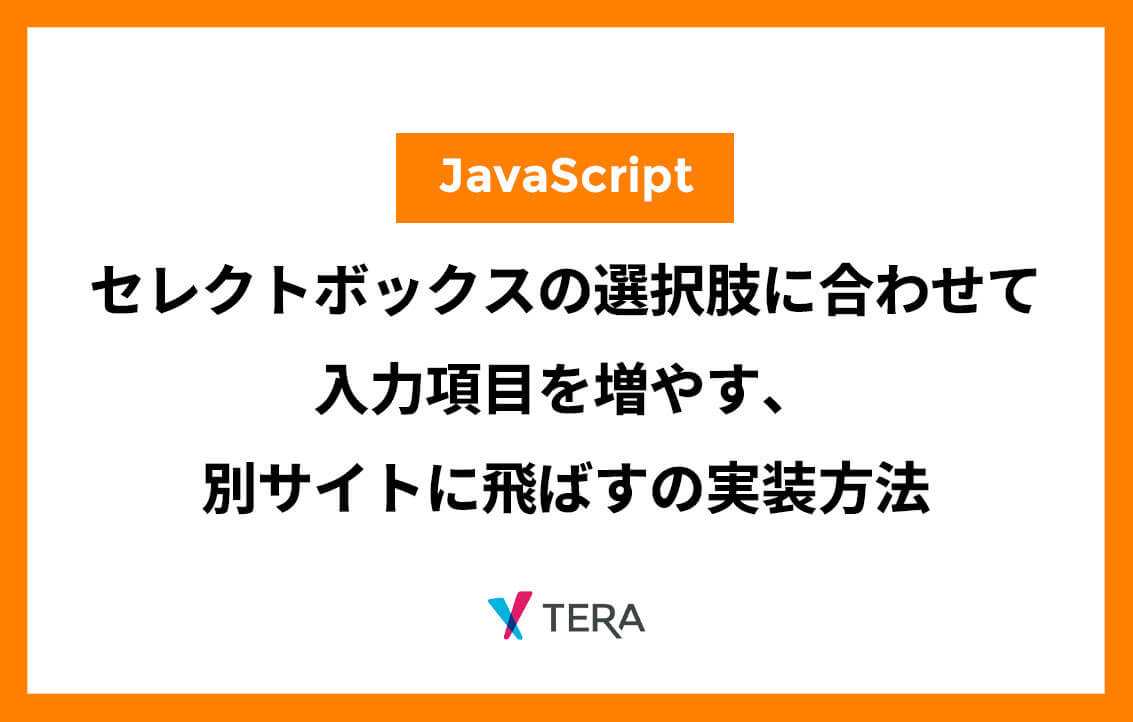
お問い合わせフォームを制作していると問い合わせの種別によって設問項目を増やしたり、別サイトや別ページに飛ばすといったことがよく発生します。
今回はJavascriptを使用して『セレクトボックスの選択肢に合わせて入力項目を増やす、別サイトに飛ばすの実装方法』を紹介します。
コードの紹介
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>お問い合わせフォーム</title> </head> <body> <form method="post" action="" enctype="multipart/form-data"> <table> <tbody> <tr> <th>お問い合わせ種別</th> <td> <select name="contact_type"> <option value="" selected="selected">選択してください</option> <option value="製品に関しての問い合わせ">製品に関しての問い合わせ</option> <option value="資料請求について">資料請求について</option> <option value="採用について">採用について</option> </select> </td> </tr> <tr data-type="product"> <th>製品を選ぶ</th> <td> <input type="checkbox" name="select_product" value="HDN-217">HDN-217 <input type="checkbox" name="select_product" value="USN-011">USN-011 <input type="checkbox" name="select_product" value="HS-10">HS-10 <input type="checkbox" name="select_product" value="IDE-12">IDE-12 </td> </tr> <tr data-type="product_text"> <th>ご自由にご記入ください。</th> <td> <textarea name="select_product_text" cols="50" rows="5" placeholder="ご記入ください。"></textarea> </td> </tr> </tbody> </table> </form> <script> document.addEventListener("DOMContentLoaded", function () { const selectBox = document.querySelector("select[name='contact_type']"); const productRow = document.querySelector("tr[data-type='product']"); const productTextRow = document.querySelector("tr[data-type='product_text']"); productRow.style.display = "none"; productTextRow.style.display = "none"; selectBox.addEventListener("change", function () { const selectedValue = this.value; productRow.style.display = "none"; productTextRow.style.display = "none"; if (selectedValue === "製品に関しての問い合わせ") { productRow.style.display = "table-row"; productTextRow.style.display = "table-row"; } else if (selectedValue === "資料請求について") { window.location.href = "https://www.yahoo.co.jp/"; } else if (selectedValue === "採用について") { window.location.href = "https://www.google.com/"; } }); }); </script> </body> </html>
コードを解説
HTML
フォームのパーツです。
セレクトボックスで『製品に関しての問い合わせ』を選んだ際に表示させる要素である『製品を選ぶ』と『ご自由にご記入ください』には、表の列であるtrタグにそれぞれデータ属性(data-type=”○○○”)を付与します。
<form method="post" action="" enctype="multipart/form-data"> <table> <tbody> <tr> <th>お問い合わせ種別</th> <td> <select name="contact_type"> <option value="" selected="selected">選択してください</option> <option value="製品に関しての問い合わせ">製品に関しての問い合わせ</option> <option value="資料請求について">資料請求について</option> <option value="採用について">採用について</option> </select> </td> </tr> <tr data-type="product"> <th>製品を選ぶ</th> <td> <input type="checkbox" name="select_product" value="HDN-217">HDN-217 <input type="checkbox" name="select_product" value="USN-011">USN-011 <input type="checkbox" name="select_product" value="HS-10">HS-10 <input type="checkbox" name="select_product" value="IDE-12">IDE-12 </td> </tr> <tr data-type="product_text"> <th>ご自由にご記入ください。</th> <td> <textarea name="select_product_text" cols="50" rows="5" placeholder="ご記入ください。"></textarea> </td> </tr> </tbody> </table> </form>
Javascript
読み込み後に実行させます。
document.addEventListener("DOMContentLoaded", function () { });
セレクトボックス、選択肢によって表示させる要素を取得していきます。
const selectBox = document.querySelector("select[name='contact_type']"); const productRow = document.querySelector("tr[data-type='product']"); const productTextRow = document.querySelector("tr[data-type='product_text']");
デフォルトでは『製品を選ぶ』と『ご自由にご記入ください』は以下で非表示にしておきます。
productRow.style.display = "none"; productTextRow.style.display = "none";
セレクトボックスを変更した場合にイベントを発火させます。
selectBox.addEventListener("change", function () { });
セレクトボックスに設定してある値を取得します。
const selectedValue = this.value;
次に選択肢を切り替えた場合に『製品を選ぶ』と『ご自由にご記入ください』を非表示にしておくための処理を書きます。
今回は3つの選択肢のうち2つは他ページのリンクへ飛ぶので不要ですが、選択肢が増えたときにこの処理を忘れてしまうので入れておきましょう。
productRow.style.display = "none"; productTextRow.style.display = "none";
セレクトボックスの値とそれぞれ一致しているかをチェックして処理を切り替えます。
一番上の『製品に関しての問い合わせ』ではスタイルシートのdisplayに『table-row』を当てて表示させます。それ以外は『window.location.href』でリダイレクトをかけています。
if (selectedValue === "製品に関しての問い合わせ") { productRow.style.display = "table-row"; productTextRow.style.display = "table-row"; } else if (selectedValue === "資料請求について") { window.location.href = "https://www.yahoo.co.jp/"; } else if (selectedValue === "採用について") { window.location.href = "https://www.google.com/"; }
以上で実装完了です。
まとめ
以上、『セレクトボックスの選択肢に合わせて入力項目を増やす、別サイトに飛ばすの実装方法』でした。
今回紹介した機能は意外に実装する場面が多いので、ぜひ参考にしてください。