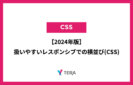
Youtubeの動画をホバーで再生させる方法
カテゴリ:WEB制作
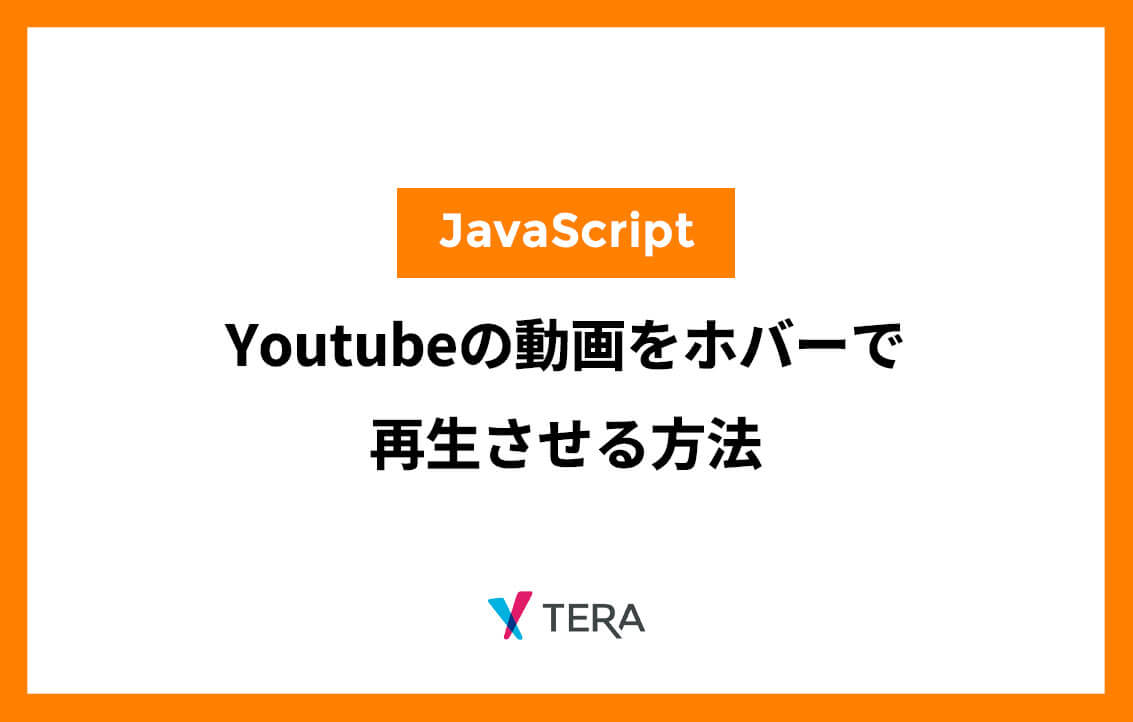
動画の素材サイトなどでよく見かけるホバーで動画が再生される機能ですが、ユーザーがクリックする必要がないこともありとても便利です。
動画クリエイターさんはポートフォリオサイトなどはとても便利でしょう。
今回は『Youtubeの動画をホバーで再生させる方法』を紹介します。
コードの紹介
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <style> ul { list-style: none; padding: 0; } li { margin-bottom: 20px; } iframe{ border: none } </style> </head> <body> <ul> <li> <iframe class="youtube" id="player1" src="https://www.youtube.com/embed/61JHONRXhjs?enablejsapi=1&mute=1"> </iframe> </li> <li> <iframe class="youtube" id="player2" src="https://www.youtube.com/embed/FprNSzG8BEY?enablejsapi=1&mute=1"> </iframe> </li> <li> <iframe class="youtube" id="player3" src="https://www.youtube.com/embed/aBoWInJXSPo?enablejsapi=1&mute=1"> </iframe> </li> <li> <iframe class="youtube" id="player4" src="https://www.youtube.com/embed/r0s5BlrbRkk?enablejsapi=1&mute=1"> </iframe> </li> <li> <iframe class="youtube" id="player5" src="https://www.youtube.com/embed/1xfZ2-2tFF8?enablejsapi=1&mute=1"> </iframe> </li> </ul> <script> const tag = document.createElement('script'); tag.src = "https://www.youtube.com/iframe_api"; const firstScriptTag = document.getElementsByTagName('script')[0]; firstScriptTag.parentNode.insertBefore(tag, firstScriptTag); const players = {}; const readyStates = {}; function onYouTubeIframeAPIReady() { const youtubeFrames = document.querySelectorAll(".youtube"); youtubeFrames.forEach((iframe) => { const playerId = iframe.id; players[playerId] = new YT.Player(playerId, { events: { onReady: () => { readyStates[playerId] = true; setupHoverEvents(playerId, players[playerId]); } } }); readyStates[playerId] = false; }); } function setupHoverEvents(playerId, player) { const iframe = document.getElementById(playerId); iframe.addEventListener("mouseenter", function () { if (readyStates[playerId]) { player.playVideo(); } }); iframe.addEventListener("mouseleave", function () { if (readyStates[playerId]) { player.pauseVideo(); } }); } </script> </body> </html>
コードの解説
HTML(iframe)
iframeにはホバー時に発火させるために『youtube』というクラス名を振っておきます。
また、マウスのオンオフでの再生・停止、他の動画がホバーされた時の再生・停止を実行するためにそれぞれにIDを振っておきます。
<iframe class="youtube" id="player1" src="https://www.youtube.com/embed/61JHONRXhjs?enablejsapi=1&mute=1"> </iframe>
src属性ですがYoutubeAPIを呼び出すため、パラメータに『?enablejsapi=1』を、自動再生を制御されないようにするために音声をミュートで再生させる『?mute=1』のパラメーターを付与します。
https://www.youtube.com/embed/ここに動画ID?enablejsapi=1&mute=1
また、上記にある『ここに動画ID』のところにYoutubeの動画IDを挿入します。動画IDは再生ページのURL内のパラメーターの『V=』のところをコピーします。
JavaScript
YouTube IFrame APIを読み込むスクリプトを動的に追加します。
const tag = document.createElement('script'); tag.src = "https://www.youtube.com/iframe_api"; const firstScriptTag = document.getElementsByTagName('script')[0]; firstScriptTag.parentNode.insertBefore(tag, firstScriptTag);
プレイヤーを管理するためのオブジェクトを設定します。
const players = {}; const readyStates = {};
YouTube IFrame APIが準備できたときに呼び出される関数を作成します。
function onYouTubeIframeAPIReady() { }
iframeに振ってある『youtube』の要素を取得します。
const youtubeFrames = document.querySelectorAll(".youtube");
ループで全てのiframeに対してYouTube Playerオブジェクトを作成、プレイヤーが準備出来た時の処理とホバーイベントの実行をさせるための関数の設定を行います。
function onYouTubeIframeAPIReady() { const youtubeFrames = document.querySelectorAll(".youtube"); youtubeFrames.forEach((iframe) => { const playerId = iframe.id; players[playerId] = new YT.Player(playerId, { events: { onReady: () => { readyStates[playerId] = true; setupHoverEvents(playerId, players[playerId]); } } }); readyStates[playerId] = false; }); }
ホバー時の再生と停止を行う関数を作成します。
function setupHoverEvents(playerId, player) { }
iframeのIDの引数をもらってiframeを取得します。
const iframe = document.getElementById(playerId);
iframeにホバーした際に再生させるための処理を書きます。
iframe.addEventListener("mouseenter", function () { if (readyStates[playerId]) { player.playVideo(); } });
ホバーを外した際の処理を書きます。
iframe.addEventListener("mouseleave", function () { if (readyStates[playerId]) { player.pauseVideo(); } });
以上で実装完了です。
再生されない場合は?
一度、その動画が非公開などになっていないかを確認してください。
非公開動画は自動再生できません。
まとめ
以上、Youtubeの動画をホバーで再生させる方法でした。